One of our customers approached us with the following issue they had with the Field Service app provided by Microsoft’s Store.
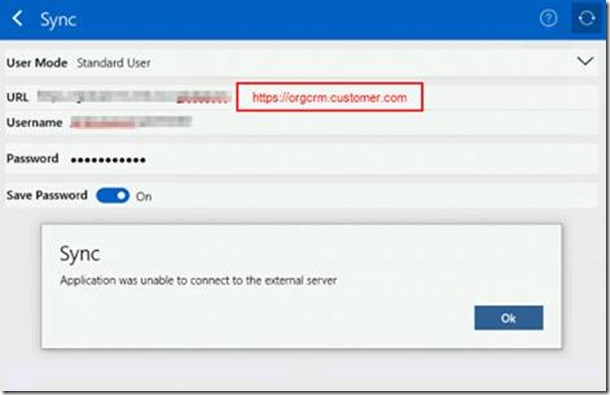
The customer has an on-premise CRM installation. Therefore the app is required to access the CRM server via ADFS in the company’s local area network.
The simplified architecture looks equal to this one:
![clip_image001[5] clip_image001[5]](https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEi2qc853VV-JLtEvloxNVZD3kDHEz2P6dFl6Pgs8zA_HpPhB036HmtwYkc42j19rr-n5p0QPEUsgnhpeYKrWnARVnTFOLIbg4ALnc3TXQgaLEk6GtSXlAg9zBQsEF93GxEsuEszKEaQyAeN/?imgmax=800)
After some time of investigation I was able to repreduce this issue with the native Resco Mobile CRM app installed via Microsoft Store with Windows 10 as well.
Whenever the machine had an internet connection the app worked just fine, but as soon as the app was launched in a local area network only without any internet connection, the app complained with the error box shown above.
Second step was to use Fiddler tracing as described here:
https://www.resco.net/fiddler-trace/
With an active internet connection I found appropriate Fiddler logs with connection requests. Interestingly, without internet connection I could not find any requests at all.
This gave me the right pointer that this is a application driven behavior and that the issue must be in the coding.
I opened a ticket with Resco support and they kindly provided me the method they use to identify an active connection in their UWP (Universal Windows Plattform) app as well as in their desktop app.
The suspicious method is:
NetworkInterface.GetIsNetworkAvailable()
https://msdn.microsoft.com/en-us/library/system.net.networkinformation.networkinterface.getisnetworkavailable(v=vs.110).aspx
Now I created two test apps, one UPW app and one regular Windows console app in Visual Studio.
I used GetIsNetworkAvailable() method in both apps and cut off any active internet connection from my test environment. Then I tried to connect to a locally hosted CRM server in our local area network.
The UWP app threw a similar exception as above whereas the console app was fully satisfied with the LAN connection.
I analysed the implementation of the GetIsNetworkAvailable method of the Universal Windows Apps.
Decompiling the System.Net.Networking.dll I found this implementation:
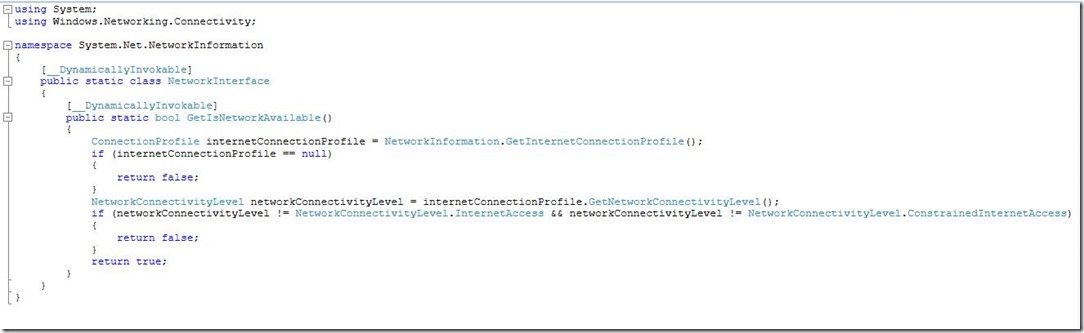
The Enum NetworkConnectivityLevel also contains „LocalAccess“.
https://docs.microsoft.com/en-us/uwp/api/windows.networking.connectivity.networkconnectivitylevel
This means in the world of UW Windows (Store) apps at this point the method GetIsNetworkAvailable() is not usable within a restricted company network.
This will make both your Resco Mobil CRM and also the MS Field Service app not usable in a company network that has the status „LocalAccess“.
Now that I knew the reason behind this problem I was able to provide the customer with a workaround described in these blogs:
https://blogs.msdn.microsoft.com/canberrapfe/2012/10/09/fake-internet-connectivity-for-your-lab-tricking-ncsi/
https://www.chrisebner.de/microsoft/windows-allgemein/network-connectivity-status-indicator-ncsi/
The customer installed a local NCSI server and pointed the desktop PC via changed registry entries to this new server which at the end made the machine think it has an active internet connection.
With that the Field Service app was usable now.
Parallel to this workaround Resco was so nice to accept my code-based workaround that they later had implemented in their UWP code base to deal with LAN networks in a better way which also enables the app to work in LAN networks:
ConnectionProfile InternetConnectionProfile = NetworkInformation.GetInternetConnectionProfile();
NetworkConnectivityLevel connection = InternetConnectionProfile.GetNetworkConnectivityLevel();
switch (connection)
{
case NetworkConnectivityLevel.None:
// return has no connection
case NetworkConnectivityLevel.LocalAccess:
case NetworkConnectivityLevel.ConstrainedInternetAccess:
case NetworkConnectivityLevel.InternetAccess :
// return has a connection
}
Since Resco Mobile CRM version 11.0.1.0 this problem is gone and was able to verify this successfully with my customer.
The customer has an on-premise CRM installation. Therefore the app is required to access the CRM server via ADFS in the company’s local area network.
The simplified architecture looks equal to this one:
After some time of investigation I was able to repreduce this issue with the native Resco Mobile CRM app installed via Microsoft Store with Windows 10 as well.
Whenever the machine had an internet connection the app worked just fine, but as soon as the app was launched in a local area network only without any internet connection, the app complained with the error box shown above.
Second step was to use Fiddler tracing as described here:
https://www.resco.net/fiddler-trace/
With an active internet connection I found appropriate Fiddler logs with connection requests. Interestingly, without internet connection I could not find any requests at all.
This gave me the right pointer that this is a application driven behavior and that the issue must be in the coding.
I opened a ticket with Resco support and they kindly provided me the method they use to identify an active connection in their UWP (Universal Windows Plattform) app as well as in their desktop app.
The suspicious method is:
NetworkInterface.GetIsNetworkAvailable()
https://msdn.microsoft.com/en-us/library/system.net.networkinformation.networkinterface.getisnetworkavailable(v=vs.110).aspx
Now I created two test apps, one UPW app and one regular Windows console app in Visual Studio.
I used GetIsNetworkAvailable() method in both apps and cut off any active internet connection from my test environment. Then I tried to connect to a locally hosted CRM server in our local area network.
The UWP app threw a similar exception as above whereas the console app was fully satisfied with the LAN connection.
I analysed the implementation of the GetIsNetworkAvailable method of the Universal Windows Apps.
Decompiling the System.Net.Networking.dll I found this implementation:
The Enum NetworkConnectivityLevel also contains „LocalAccess“.
https://docs.microsoft.com/en-us/uwp/api/windows.networking.connectivity.networkconnectivitylevel
This means in the world of UW Windows (Store) apps at this point the method GetIsNetworkAvailable() is not usable within a restricted company network.
This will make both your Resco Mobil CRM and also the MS Field Service app not usable in a company network that has the status „LocalAccess“.
Now that I knew the reason behind this problem I was able to provide the customer with a workaround described in these blogs:
https://blogs.msdn.microsoft.com/canberrapfe/2012/10/09/fake-internet-connectivity-for-your-lab-tricking-ncsi/
https://www.chrisebner.de/microsoft/windows-allgemein/network-connectivity-status-indicator-ncsi/
The customer installed a local NCSI server and pointed the desktop PC via changed registry entries to this new server which at the end made the machine think it has an active internet connection.
With that the Field Service app was usable now.
Parallel to this workaround Resco was so nice to accept my code-based workaround that they later had implemented in their UWP code base to deal with LAN networks in a better way which also enables the app to work in LAN networks:
ConnectionProfile InternetConnectionProfile = NetworkInformation.GetInternetConnectionProfile();
NetworkConnectivityLevel connection = InternetConnectionProfile.GetNetworkConnectivityLevel();
switch (connection)
{
case NetworkConnectivityLevel.None:
// return has no connection
case NetworkConnectivityLevel.LocalAccess:
case NetworkConnectivityLevel.ConstrainedInternetAccess:
case NetworkConnectivityLevel.InternetAccess :
// return has a connection
}
Since Resco Mobile CRM version 11.0.1.0 this problem is gone and was able to verify this successfully with my customer.
Comments
Post a Comment